Newer
Older

Arthur Le Bars
committed
#!/usr/bin/env python3

Arthur Le Bars
committed
import bioblend
import bioblend.galaxy.objects
import argparse
import os
import logging
import sys

Arthur Le Bars
committed
import json

Arthur Le Bars
committed
import time

Arthur Le Bars
committed

Arthur Le Bars
committed
from bioblend import galaxy

Arthur Le Bars
committed
import utilities
import speciesData
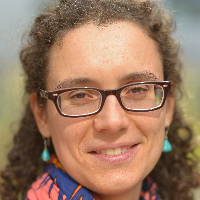
Loraine Gueguen
committed
import constants
import phaoexplorer_constants

Arthur Le Bars
committed

Arthur Le Bars
committed
"""

Arthur Le Bars
committed

Arthur Le Bars
committed
Usage: $ python3 gga_init.py -i input_example.yml --config [config file] [OPTIONS]

Arthur Le Bars
committed
"""

Arthur Le Bars
committed
"""
Run a workflow into the galaxy instance's history of a given species

Arthur Le Bars
committed

Arthur Le Bars
committed
This script is made to work for a Phaeoexplorer-specific workflow, but can be adapted to run any workflow,

Arthur Le Bars
committed
provided the user creates their own workflow in a .ga format, and change the set_parameters function

Arthur Le Bars
committed
to have the correct parameters for their workflow

Arthur Le Bars
committed

Arthur Le Bars
committed
"""
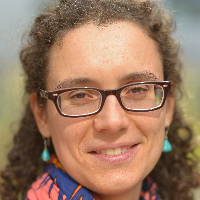
Loraine Gueguen
committed
def __init__(self, parameters_dictionary):
super().__init__(parameters_dictionary)
self.chado_species_name = " ".join(utilities.filter_empty_not_empty_items(
[self.species, self.strain, self.sex])["not_empty"])
self.abbreviation = self.genus_uppercase[0] + ". " + self.chado_species_name
self.common = self.name
if not self.common_name is None and self.common_name != "":
self.common = self.common_name
self.history_name = str(self.genus_species)
self.genome_analysis_name = "genome v{0} of {1}".format(self.genome_version, self.full_name)
self.genome_analysis_programversion = "genome v{0}".format(self.genome_version)
self.genome_analysis_sourcename = self.full_name
self.ogs_analysis_name = "OGS{0} of {1}".format(self.ogs_version, self.full_name)
self.ogs_analysis_programversion = "OGS{0}".format(self.ogs_version)
self.ogs_analysis_sourcename = self.full_name
def set_history(self):

Arthur Le Bars
committed
"""

Arthur Le Bars
committed
Create or set the working history to the current species one

Arthur Le Bars
committed
:return:
"""

Arthur Le Bars
committed
try:
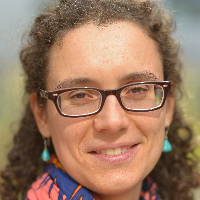
Loraine Gueguen
committed
histories = self.instance.histories.get_histories(name=self.history_name)

Arthur Le Bars
committed
self.history_id = histories[0]["id"]
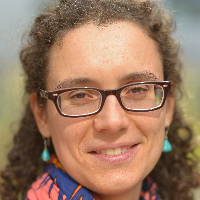
Loraine Gueguen
committed
logging.debug("History ID set for {0}: {1}".format(self.history_name, self.history_id))

Arthur Le Bars
committed
except IndexError:
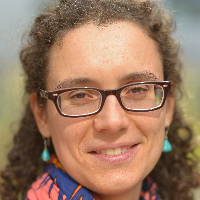
Loraine Gueguen
committed
logging.info("Creating history for %s" % self.history_name)
history = self.instance.histories.create_history(name=self.history_name)
self.history_id = history["id"]
logging.debug("History ID set for {0}: {1}".format(self.history_name, self.history_id))

Arthur Le Bars
committed
return self.history_id
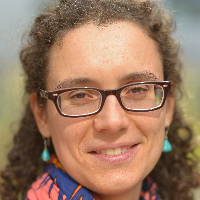
Loraine Gueguen
committed
def set_galaxy_instance(self):

Arthur Le Bars
committed
"""
Test the connection to the galaxy instance for the current organism
Exit if we cannot connect to the instance
"""
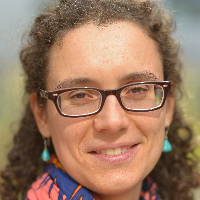
Loraine Gueguen
committed
logging.debug("Connecting to the galaxy instance (%s)" % self.instance_url)

Arthur Le Bars
committed
self.instance = galaxy.GalaxyInstance(url=self.instance_url,
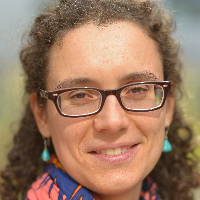
Loraine Gueguen
committed
email=self.config[constants.CONF_GALAXY_DEFAULT_ADMIN_EMAIL],
password=self.config[constants.CONF_GALAXY_DEFAULT_ADMIN_PASSWORD]

Arthur Le Bars
committed
)
try:
self.instance.histories.get_histories()
except bioblend.ConnectionError:
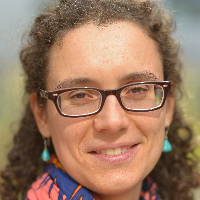
Loraine Gueguen
committed
logging.critical("Cannot connect to galaxy instance (%s) " % self.instance_url)

Arthur Le Bars
committed
sys.exit()
else:
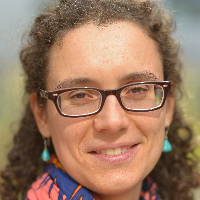
Loraine Gueguen
committed
logging.debug("Successfully connected to galaxy instance (%s) " % self.instance_url)
def install_changesets_revisions_for_individual_tools(self):

Arthur Le Bars
committed
"""
This function is used to verify that installed tools called outside workflows have the correct versions and changesets
If it finds versions don't match, will install the correct version + changeset in the instance
Doesn't do anything if versions match
:return:
"""
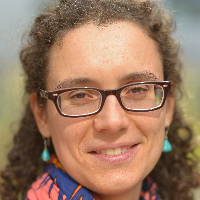
Loraine Gueguen
committed
self.set_galaxy_instance()
logging.info("Validating installed individual tools versions and changesets")

Arthur Le Bars
committed
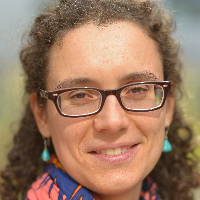
Loraine Gueguen
committed
# Verify that the add_organism and add_analysis versions are correct in the instance
add_organism_tool = self.instance.tools.show_tool(phaoexplorer_constants.ADD_ORGANISM_TOOL_ID)
add_analysis_tool = self.instance.tools.show_tool(phaoexplorer_constants.ADD_ANALYSIS_TOOL_ID)
get_organisms_tool = self.instance.tools.show_tool(phaoexplorer_constants.GET_ORGANISMS_TOOL_ID)
get_analyses_tool = self.instance.tools.show_tool(phaoexplorer_constants.GET_ANALYSES_TOOL_ID)
analysis_sync_tool = self.instance.tools.show_tool(phaoexplorer_constants.ANALYSIS_SYNC_TOOL_ID)
organism_sync_tool = self.instance.tools.show_tool(phaoexplorer_constants.ORGANISM_SYNC_TOOL_ID)
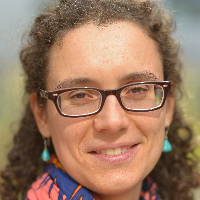
Loraine Gueguen
committed
# changeset for 2.3.4+galaxy0 has to be manually found because there is no way to get the wanted changeset of a non installed tool via bioblend
# except for workflows (.ga) that already contain the changeset revisions inside the steps ids

Arthur Le Bars
committed
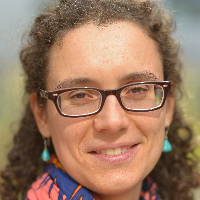
Loraine Gueguen
committed
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
utilities.install_repository_revision(current_version=get_organisms_tool["version"],
toolshed_dict=get_organisms_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.GET_ORGANISMS_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.GET_ORGANISMS_TOOL_CHANGESET_REVISION,
instance=self.instance)
utilities.install_repository_revision(current_version=get_analyses_tool["version"],
toolshed_dict=get_analyses_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.GET_ANALYSES_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.GET_ANALYSES_TOOL_CHANGESET_REVISION,
instance=self.instance)
utilities.install_repository_revision(current_version=add_organism_tool["version"],
toolshed_dict=add_organism_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.ADD_ORGANISM_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.ADD_ORGANISM_TOOL_CHANGESET_REVISION,
instance=self.instance)
utilities.install_repository_revision(current_version=add_analysis_tool["version"],
toolshed_dict=add_analysis_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.ADD_ANALYSIS_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.ADD_ANALYSIS_TOOL_CHANGESET_REVISION,
instance=self.instance)
utilities.install_repository_revision(current_version=analysis_sync_tool["version"],
toolshed_dict=analysis_sync_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.ANALYSIS_SYNC_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.ANALYSIS_SYNC_TOOL_CHANGESET_REVISION,
instance=self.instance)
utilities.install_repository_revision(current_version=organism_sync_tool["version"],
toolshed_dict=organism_sync_tool["tool_shed_repository"],
version_to_install=phaoexplorer_constants.ORGANISM_SYNC_TOOL_VERSION,
changeset_revision=phaoexplorer_constants.ORGANISM_SYNC_TOOL_CHANGESET_REVISION,
instance=self.instance)

Arthur Le Bars
committed

Arthur Le Bars
committed
logging.info("Success: individual tools versions and changesets validated")
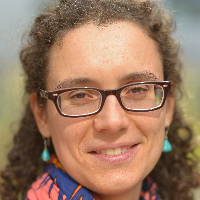
Loraine Gueguen
committed
def add_analysis(self, name, programversion, sourcename):
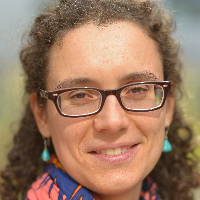
Loraine Gueguen
committed
add_analysis_tool_dataset = utilities.run_tool_and_download_single_output_dataset(
instance=self.instance,
tool_id=phaoexplorer_constants.ADD_ANALYSIS_TOOL_ID,
history_id=self.history_id,
tool_inputs={"name": name,
"program": phaoexplorer_constants.ADD_ANALYSIS_TOOL_PARAM_PROGRAM,
"programversion": programversion,
"sourcename": sourcename,
"date_executed": phaoexplorer_constants.ADD_ANALYSIS_TOOL_PARAM_DATE})
analysis_dict = json.loads(add_analysis_tool_dataset)
analysis_id = str(analysis_dict["analysis_id"])
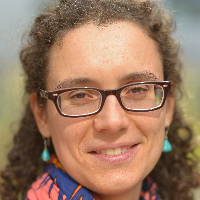
Loraine Gueguen
committed
return analysis_id

Arthur Le Bars
committed
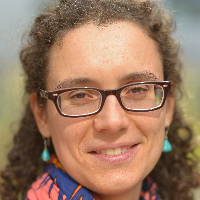
Loraine Gueguen
committed
def sync_analysis(self, analysis_id):

Arthur Le Bars
committed
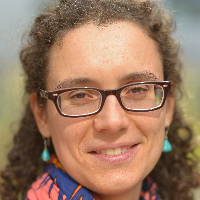
Loraine Gueguen
committed
time.sleep(60)
utilities.run_tool(
instance=self.instance,
tool_id=phaoexplorer_constants.ANALYSIS_SYNC_TOOL_ID,
history_id=self.history_id,
tool_inputs={"analysis_id": analysis_id})

Arthur Le Bars
committed
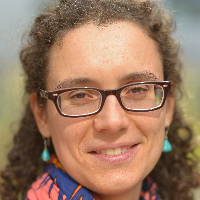
Loraine Gueguen
committed
def add_organism_and_sync(self):
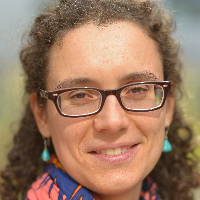
Loraine Gueguen
committed
get_organisms_tool_dataset = utilities.run_tool_and_download_single_output_dataset(
instance=self.instance,
tool_id=phaoexplorer_constants.GET_ORGANISMS_TOOL_ID,

Arthur Le Bars
committed
history_id=self.history_id,
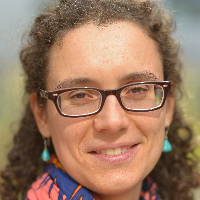
Loraine Gueguen
committed
tool_inputs={},
time_sleep=10
)
organisms_dict_list = json.loads(get_organisms_tool_dataset) # Turn the dataset into a list for parsing
Loading
Loading full blame...